It’s crucial to check page load speed since it is not only a key parameter that impacts the user experience with your website but is also one of the most important determinants of your rankings. Google armed webmasters and SEOs with the PageSpeed Insights tool that checks page load speed and evaluates the website performance on both mobile and desktop devices as well as recommends the set of improvements for any given page to help it load faster.
By all means, it’s a great tool. However, if you need to constantly monitor the performance of your pages to see the impact of the changes that either you or search engines have recently implemented, your page speed checks should be ongoing. This is where the inconvenience of manually pasting one link at a time begins to show. If you need to check a few dozens of links of a certain domain, it is a pretty time-consuming process especially if you have more than one website on your hands.
At Webxloo, we put a lot of effort into automating repetitive jobs of any kind that’s why we have created a bulk page speed checker script that utilizes Google’s PageSpeed Insights API.
The purpose of this article is to educate you on how to set everything up, run the script and automate the ongoing page speed checks to save you valuable time. Follow the steps below and don’t hesitate to contact us in case of any questions along the way.
1. Go to API Console.
2. Create a new project:
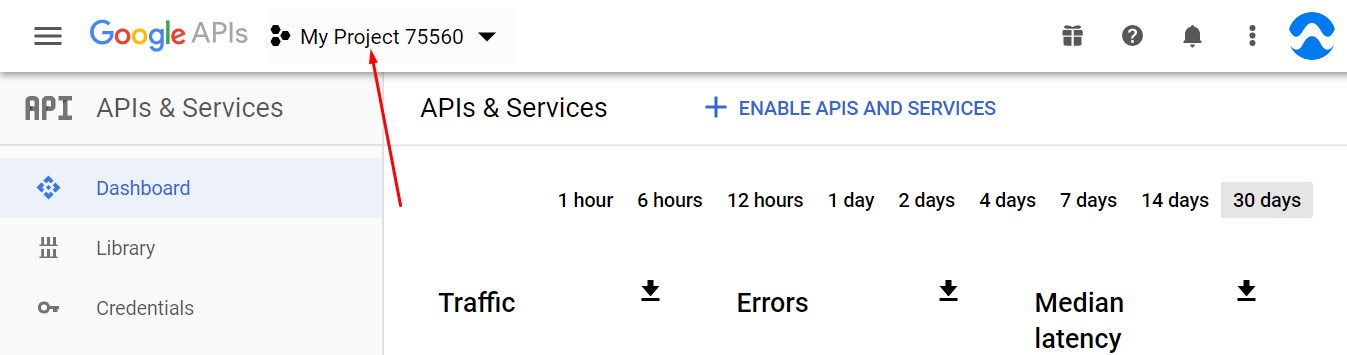
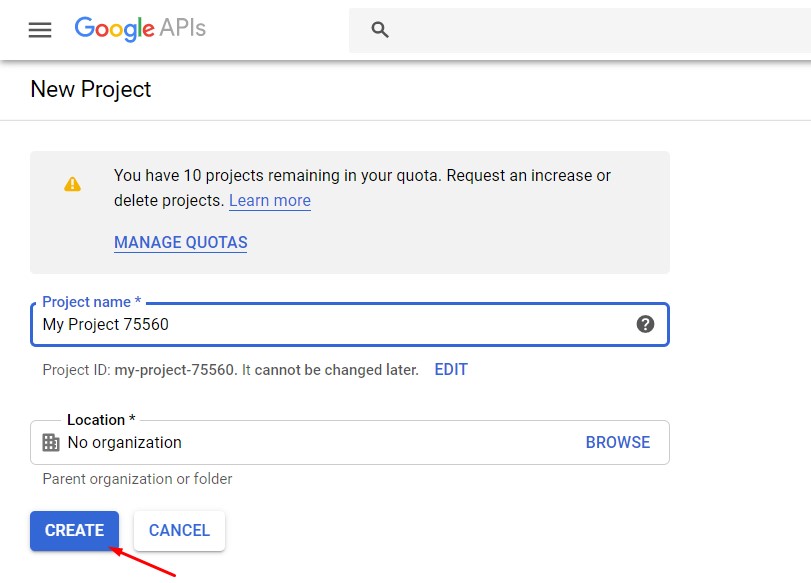
3. Go to ‘Library’ on the left side navigation and search for ‘PageSpeed Insights API’. Click ‘Enable’ once you’ve found it:
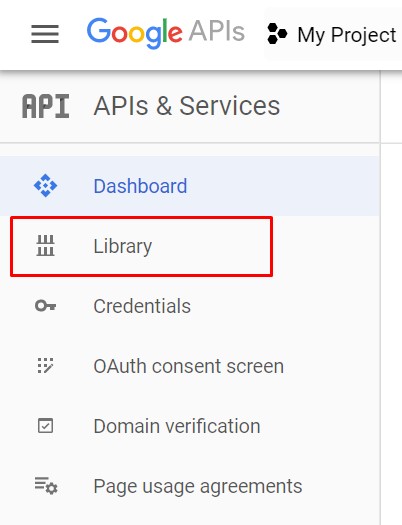
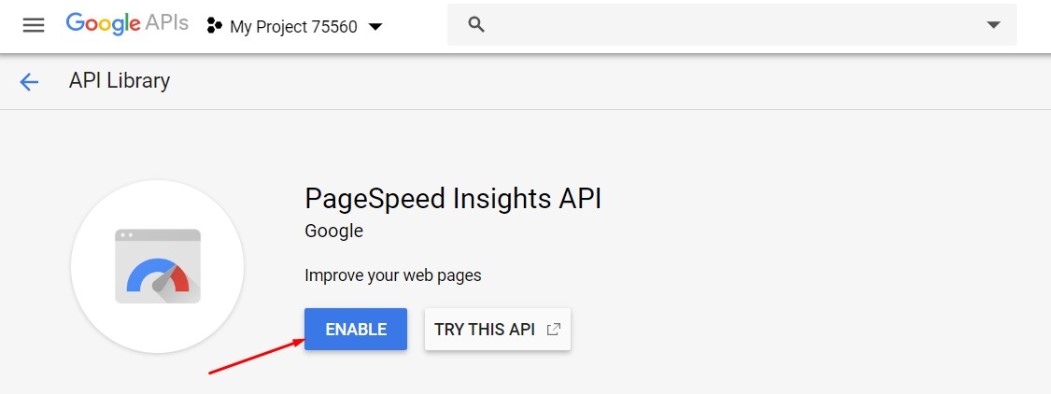
4. Go to ‘Credentials’. Click on ‘Create Credentials’ and then select ‘API Key’:
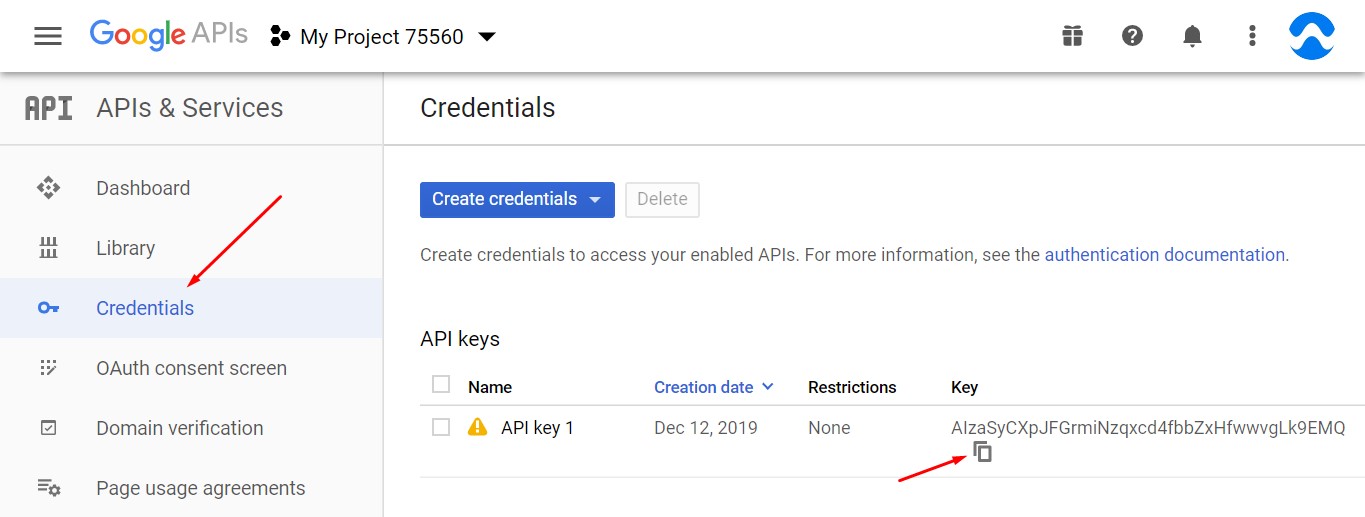
5. Go to Google Sheets within your Google account and create a new spreadsheet, name it accordingly. For example, Daily Bulk Page Speed Check. Go to ‘Tools’ and click on a ‘Script editor’:
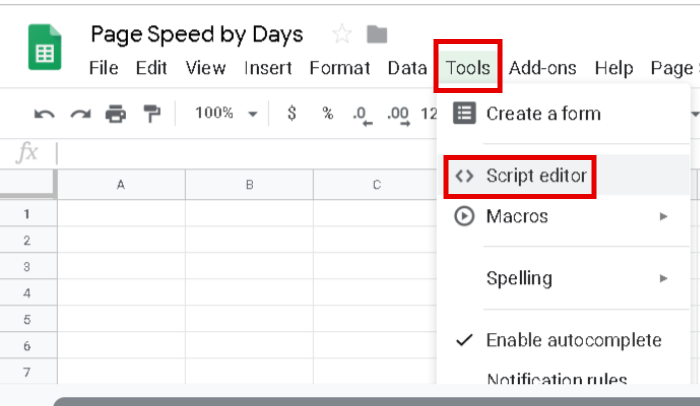
6. You will be taken to a new tab. Delete the default text:
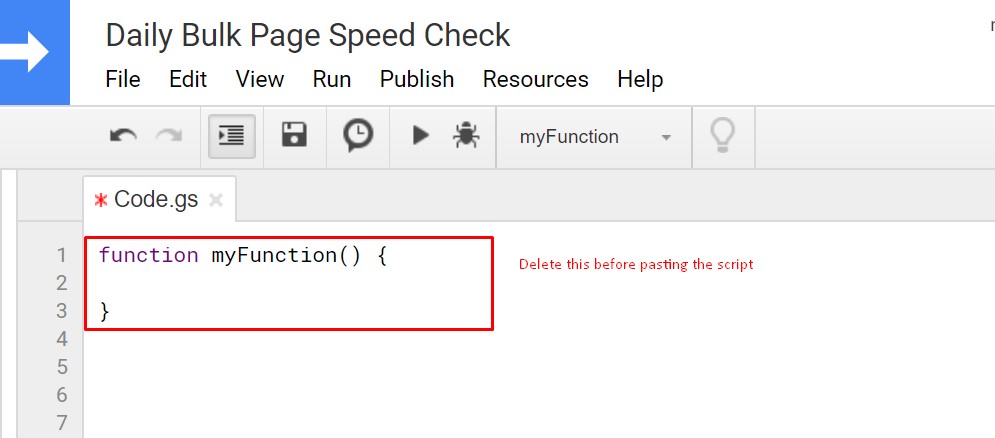
7. Paste the whole script into the tab:
//------------------------------------ UI Functions -----------------------------------------------// function onOpen() { SpreadsheetApp.getUi() .createMenu('Page Speed') .addItem('Run', 'run') //if you need to check more than 10 sites you should copy-paste this row and the run function .addToUi(); } //--------------------------------------- Schedule ------------------------------------------------// function autoTrigger(){ ScriptApp.newTrigger('run') //if you add extra functions for extra URLs, you should copy this trigger .timeBased().atHour(1) .everyDays(1) .create(); } //------------------------------------ End of Schedule --------------------------------------------// //------------------------------------- Run Functions ---------------------------------------------// function run() { header(); var url = []; url = read('A2','A37'); //change data range (URLs on sheet Config) GetPageSpeed(url,0,6); // change second and third parameters with row numbers of URLs (from to) } //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------- Get Mob & Desk Page Speed -------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// function GetPageSpeed(url,start_i,finish_i){ if(url){ var res_mob = []; var mob = []; var res_desk = []; var ThreeG = []; for(var i=start_i;i<finish_i;i++){ var current_url = url[i]; res_mob[i] = GetPageSpeedMobPlusThreeG(current_url); mob[i] = res_mob[i].score * 100; res_desk[i] = GetPageSpeedDesk(current_url) * 100; ThreeG[i] = res_mob[i].ThreeG; write(mob[i].toFixed(0),res_desk[i].toFixed(0),parseInt(ThreeG[i],10),current_url,0); shift=0; } } } //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //------------------------------------------------------- End of Get Page Speed ----------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //------------------------------------ Work Functions ---------------------------------------------// //------------------------------------ Read URLs from Sheet 'Config' ------------------------------// function read(start,end){ var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("Config"); if (sheet == null) { SpreadsheetApp.getUi().alert("File Config does not exist"); return false; } else { var data = sheet.getRange(start+':'+end).getValues(); return data; } } //------------------------------------ End of Read URLs -------------------------------------------// //------------------------------------ Header for Result Sheet ------------------------------------// function header(){ var date = cur_date(); var spreadsheet = SpreadsheetApp.getActiveSpreadsheet(); if(spreadsheet.getSheetByName(date)){ SpreadsheetApp.getUi().alert("Today's report already exists"); return false; } SpreadsheetApp.getActiveSpreadsheet().insertSheet(date); var spreadsheet = SpreadsheetApp.getActiveSpreadsheet(); SpreadsheetApp.setActiveSheet(spreadsheet.getSheetByName(date)); var sheet = SpreadsheetApp.getActiveSheet(); sheet.appendRow(['URL','Mobile','Desktop','3G']); return true; } //------------------------------------ End of Header ----------------------------------------------// //------------------------------------ Write Result -----------------------------------------------// function write(res_mob,res_desk,ThreeG,url,shift) { var date = cur_date(); var spreadsheet = SpreadsheetApp.getActiveSpreadsheet(); SpreadsheetApp.setActiveSheet(spreadsheet.getSheetByName(date)); var sheet = SpreadsheetApp.getActiveSheet(); var columns = sheet.getRange('B2:D'); columns.setNumberFormat("##"); for(var i=0;i<shift;i++){ sheet.appendRow([" "]); } sheet.appendRow([url.toString(),res_mob,res_desk,ThreeG]); } //------------------------------------ End of Write Result ----------------------------------------// //----------------------------------------- Current Date ------------------------------------------// function cur_date() { var date = Utilities.formatDate(new Date(), "GMT+1", "dd/MM/yyyy"); return date; } //-------------------------------------- End of Current Date --------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //----------------------------------------------------------------------- Page Speed --------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// function GetPageSpeedDesk(url){ var url_local = 'https://www.googleapis.com/pagespeedonline/v5/runPagespeed?url='+url.toString()+'&category=performance&strategy=desktop&key=YOUR_KEY'; //replace YOUR_KEY with your API key var options = {muteHttpExceptions: true}; var resData = UrlFetchApp.fetch(url_local,options); // response from server (string) var resDataJson = JSON.parse(resData); // parse in json-object if(resData.getResponseCode() == "200") return resDataJson.lighthouseResult.categories.performance.score; else{ return resData.getResponseCode(); MailApp.sendEmail("YOUR_MAIL", //replace YOUR_MAIL with mail where you want to get error messages "Page Speed App - Problem URL", "Can`t get Page Speed for"+url+". Response from server is" + resData.getResponseCode()); } } function GetPageSpeedMobPlusThreeG(url){ var url_local = 'https://www.googleapis.com/pagespeedonline/v5/runPagespeed?url='+url.toString()+'&category=performance&strategy=mobile&key=YOUR_KEY'; //replace YOUR_KEY with your API key var options = {muteHttpExceptions: true}; var resData = UrlFetchApp.fetch(url_local,options); // response from server (string) var resDataJson = JSON.parse(resData); // parse in json-object if(resData.getResponseCode() == "200"){ var score = resDataJson.lighthouseResult.categories.performance.score; var ThreeG = resDataJson.lighthouseResult.audits['first-contentful-paint-3g'].displayValue; var result = { score: score, ThreeG: ThreeG} return result;} else{ return resData.getResponseCode(); MailApp.sendEmail("YOUR_MAIL", //replace YOUR_MAIL with mail where you want to get error messages "Page Speed App - Problem URL", "Can`t get Page Speed for"+url+". Response from server is" + resData.getResponseCode()); } } //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //---------------------------------------------------------------------- End of Page Speed --------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------// //-------------------------------------------------------------------------------------------------------------------------------------------------------------------------//
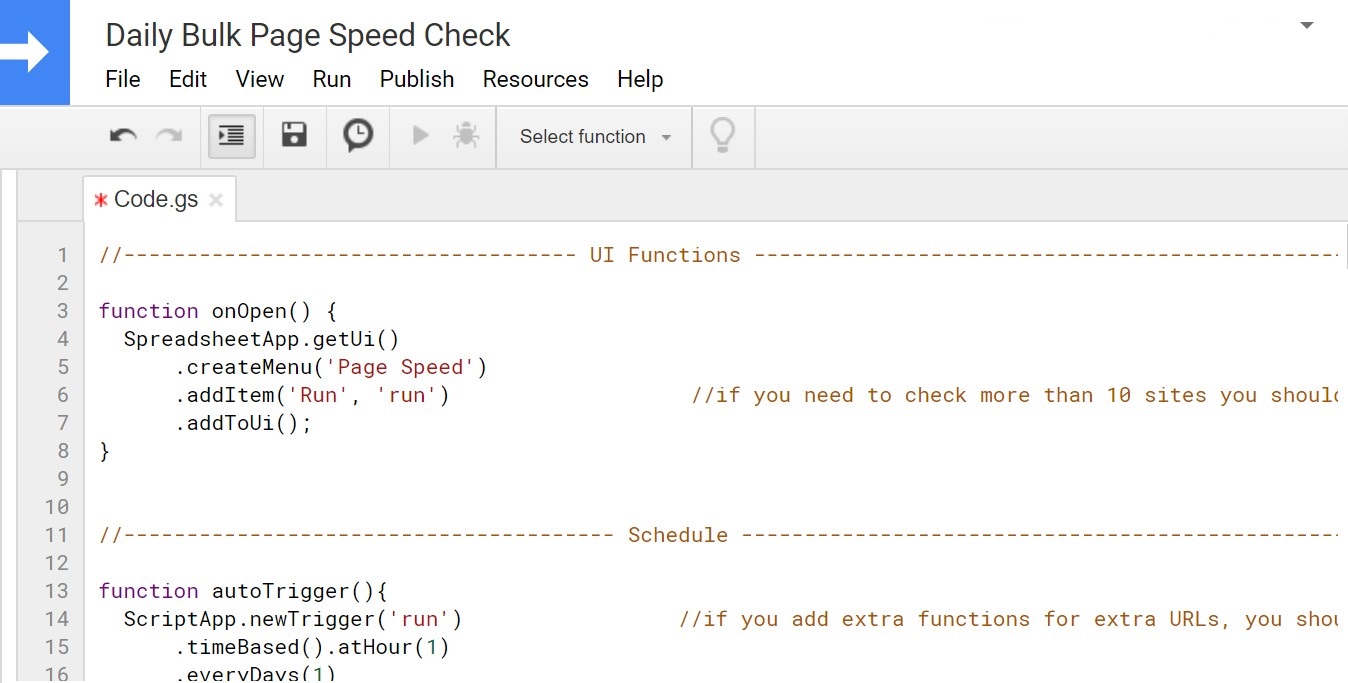
8. Now you need to insert your API Key instead of the default values. To do that, go back to API Console, click on ‘Credentials’ and copy your own API Key using a Copy File icon:
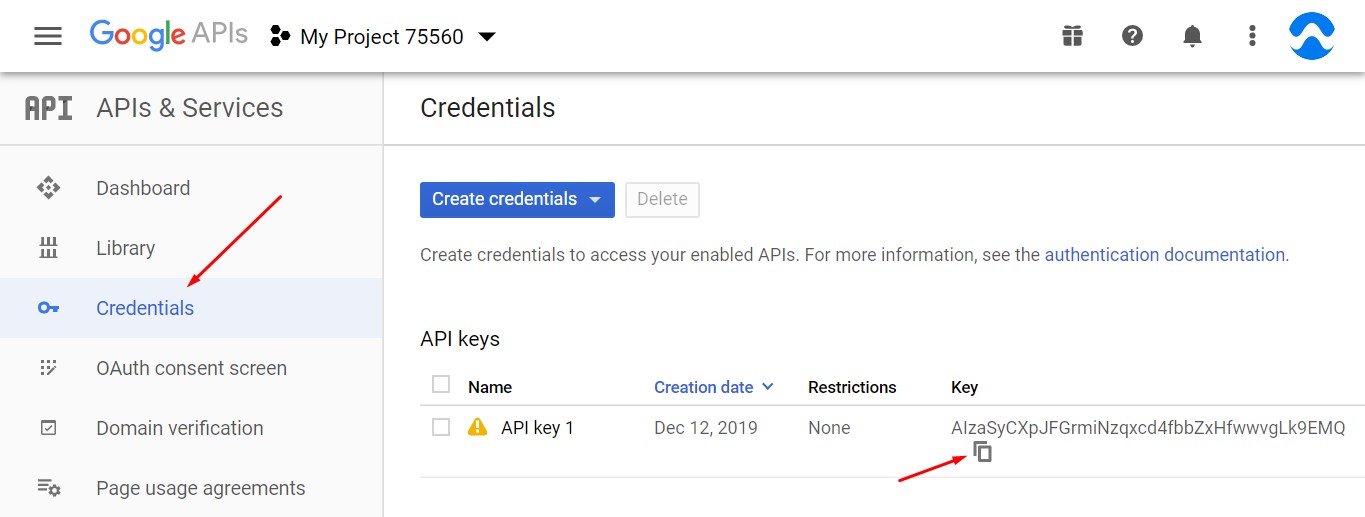
9. Go back to the script editor and paste the API Key that you’ve just copied instead of ‘YOUR KEY’ values:
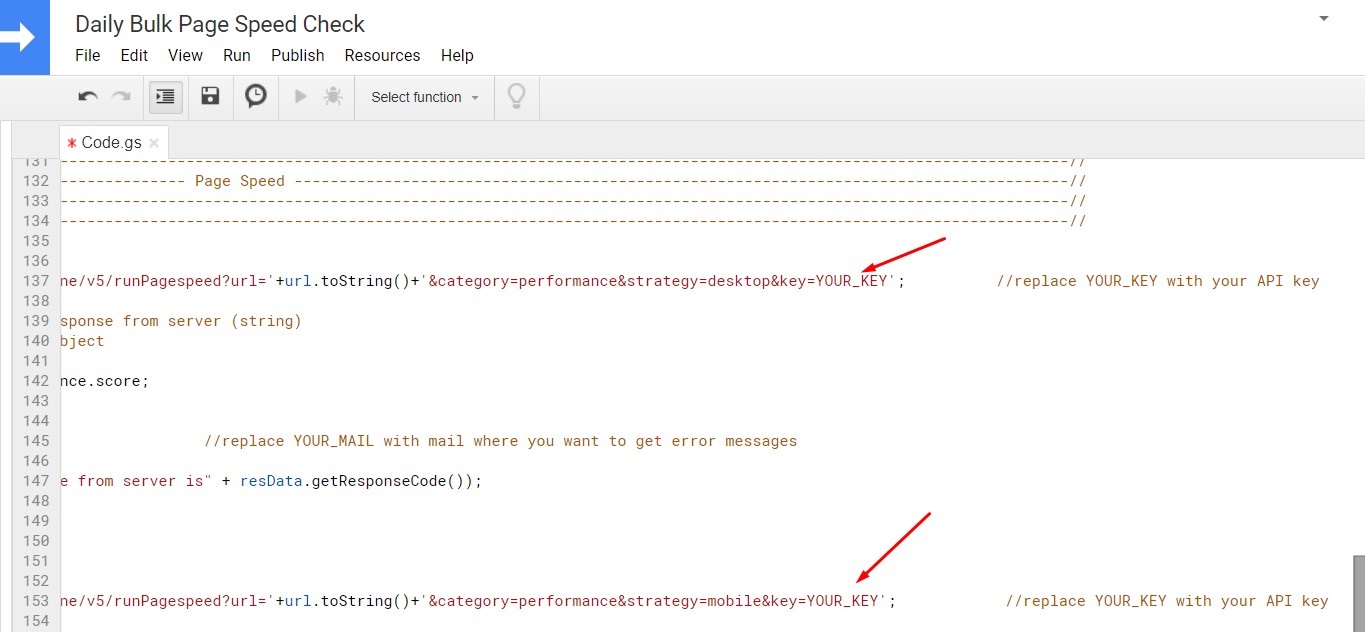
To specify the email address where you would like to get any error messages to, replace ‘YOUR MAIL’ with a valid email address:
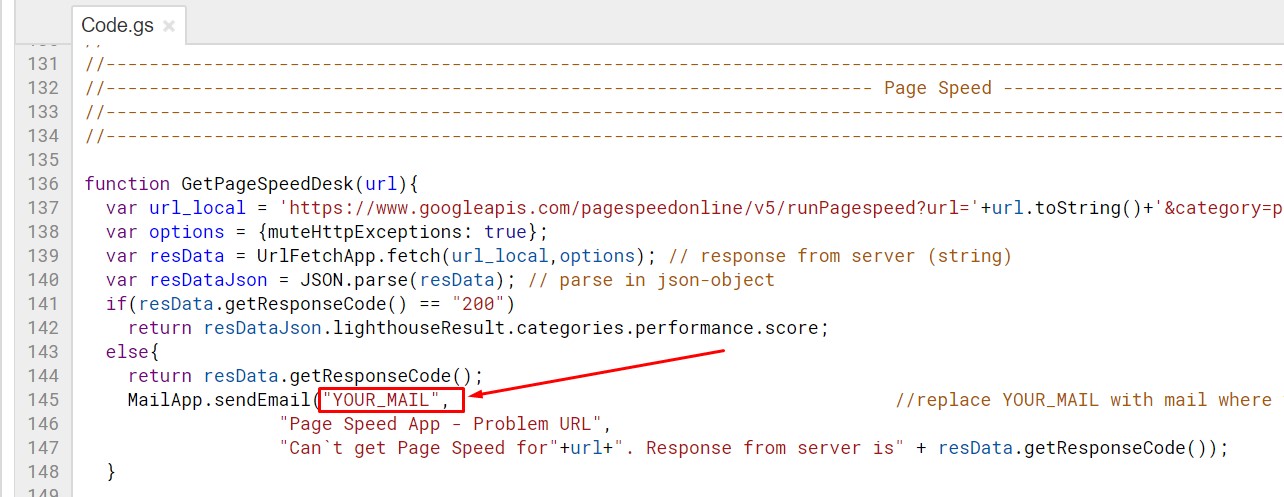
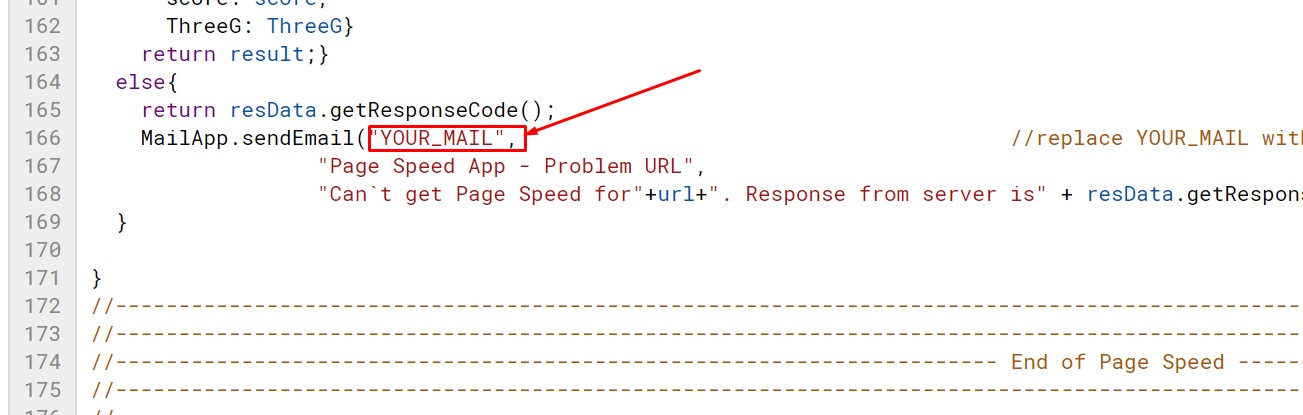
10. Save the script via the File tab or simply by clicking on a Floppy Disk icon.
11. Go back to the spreadsheet from #5 and refresh the page. You will see that a new ‘Page Speed’ tab has been added to the menu bar:
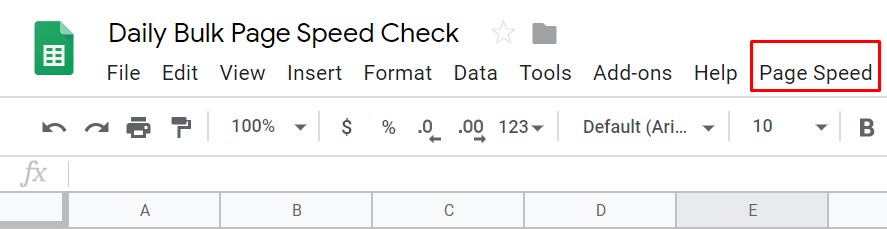
12. Add a new sheet and name it ‘Config’. Copy the links* you want to check and past them to this sheet:
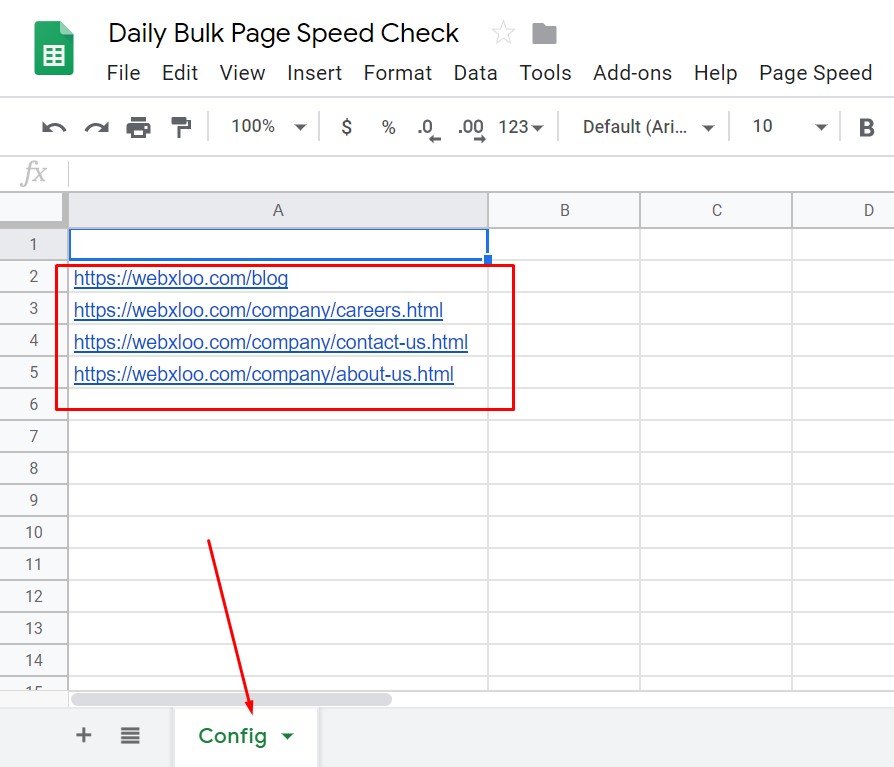
As an example, we will check four of our links. Please note that you need to leave the first row empty for a table header.
*Note: The maximum time allowed for script execution is 6 minutes. The number of URLs that can be checked during this time ranges from 7 to 10. If you need to check more than 10 URLs at a time, you should amend the script a bit:
- add a new row as it’s shown on a screenshot:
.addItem('Run1', 'run1')
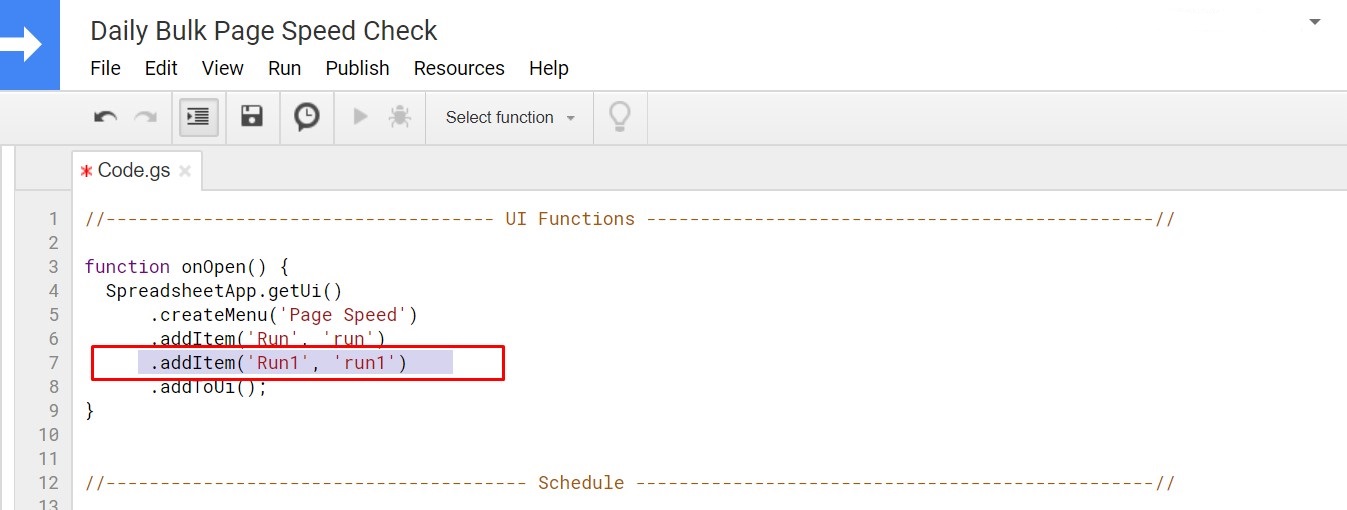
- add one more run function and name it Run1:
function run1() {
var url = [];
url = read('A2','A37'); //change data range (URLs on sheet Config)
GetPageSpeed(url,0,4); // change second and third parameters with row numbers of URLs (from to)
}
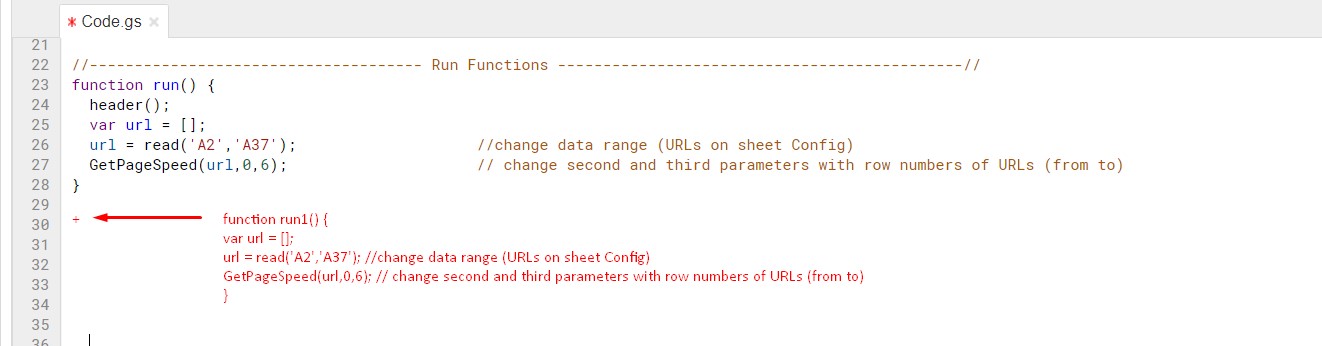
13. Before proceeding to run a script, specify the row numbers of URLs you want to check. For our four links, since the blank row is zero row, the numbering starts at 0 and ends at 4:
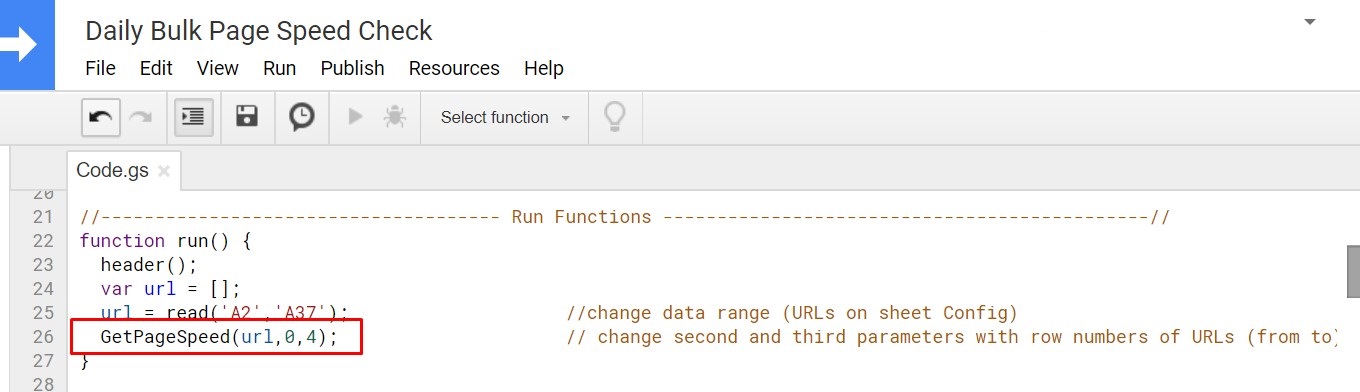
14. Now we are all set to run the script and get the fruits of our labors. Go to your Google sheet, click on a Page Speed tab and click ‘Run’, then click ‘Continue’:
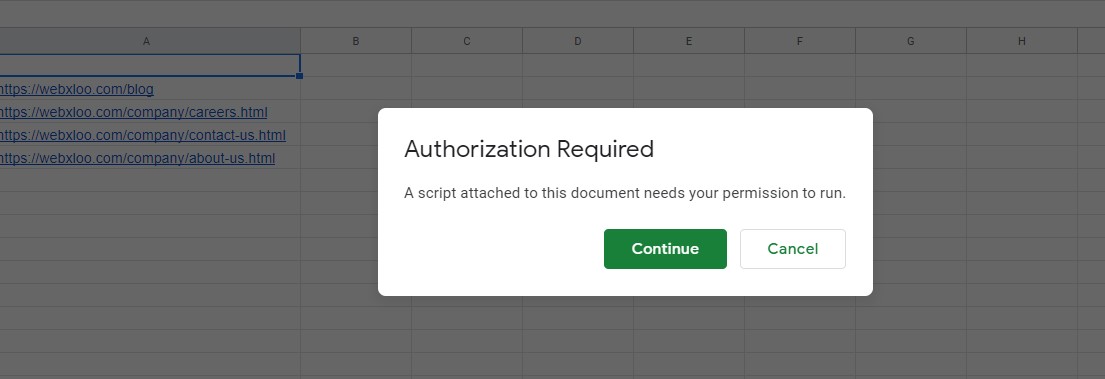
Click ‘Advanced’:
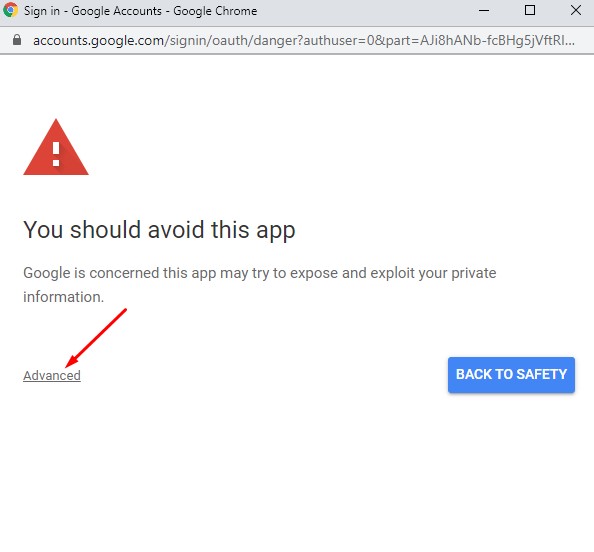
Click ‘Go to Daily Bulk Page Speed Check’ (or whatever your Google spreadsheet with URLs is named):
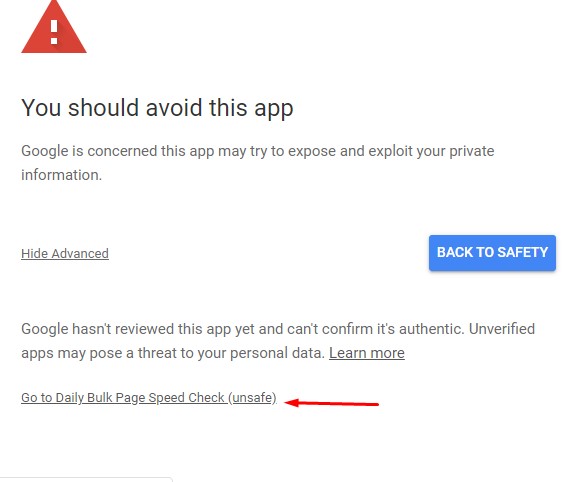
Click Allow. You will be then taken to the spreadsheet and see the ‘Running script’ notification.
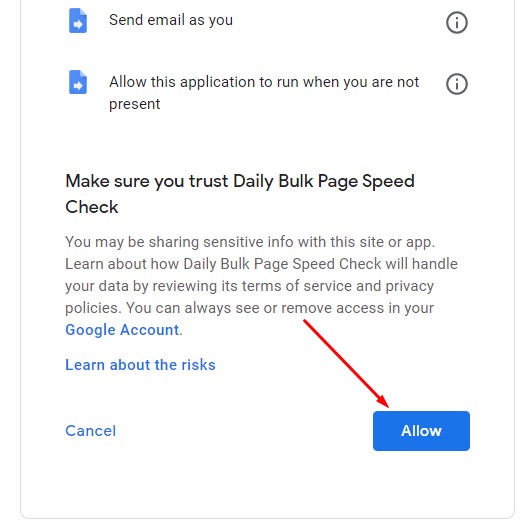
15. Once the page speed check is finished, you will see the neatly displayed Page Speed scores on desktops and mobile devices along with the time needed to load your page on a 3G network in milliseconds. We have included this parameter as 3G is one of the dominant networks worldwide and a lot of users are browsing the Internet via this mobile technology:
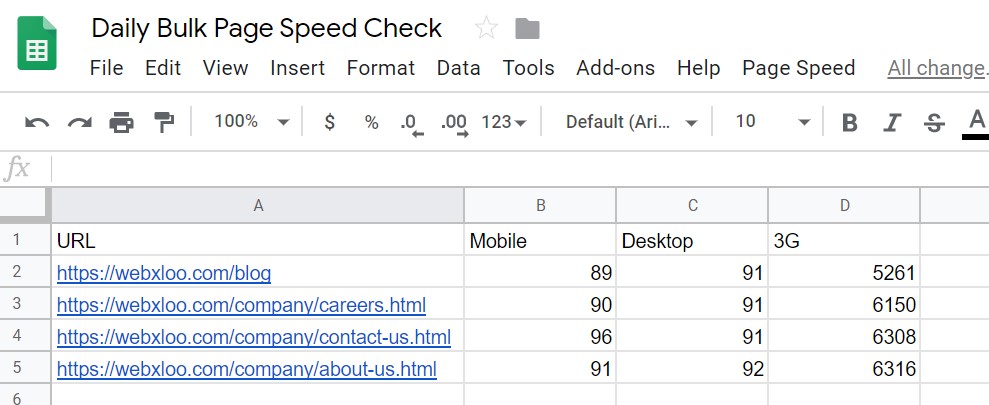
Now you know how to check page load speed for multiple URLs at once. If any questions arise during the setup process or while running the script, do not hesitate to contact us. Stay tuned to our blog for more SEO tutorials.
Last updated on July 18th, 2023